6 minutes
A Beginner’s Guide to Smart Contract Programming on the Flow Blockchain with Cadence
In this article, we will provide a crash course on Cadence and smart contract programming on the Flow blockchain. We will cover the basics of Cadence and explain how to write smart contracts on the Flow network.
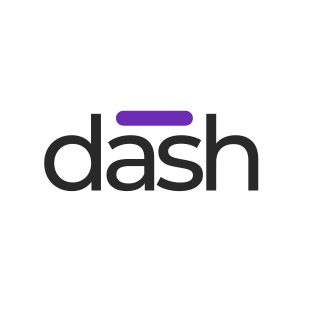
Purple Dash
27/09/2023 8:44 AMTable of Contents
Smart contract programming has gained immense popularity in the recent past, primarily due to the growing demand for decentralized applications (DApps). Smart contracts are self-executing agreements that run on a blockchain network. One of the most popular blockchain networks that allow for smart contract programming is the Ethereum network. However, with the growing demand for more scalable and efficient blockchain solutions, new blockchain networks, such as Flow, are emerging.
Flow is a blockchain network that was designed specifically for DApps and digital assets. It was created by Dapper Labs, the creators of CryptoKitties, and has been gaining popularity in the NFT space. Flow is built on a unique programming language called Cadence, which was developed to make smart contract programming more accessible and secure.
In this article, we will provide a crash course on Cadence and smart contract programming on the Flow blockchain. We will cover the basics of Cadence and explain how to write smart contracts on the Flow network. We will also discuss the benefits of using Flow for DApps and digital assets.
What is Cadence?
Cadence is a safe and secure programming language that was designed specifically for smart contracts and DApps on the Flow blockchain. It is a resource-oriented language that focuses on improving the security and efficiency of smart contract programming. Cadence uses a unique syntax that is easy to read and understand, even for non-programmers.
One of the main features of Cadence is resource-oriented programming. In resource-oriented programming, resources are the primary objects that are manipulated by the program. Resources are objects that have state and can be owned by an account. Cadence also supports the use of reference types, which are objects that are not owned by any account and can be shared between different resources.
Another important feature of Cadence is its type system. Cadence has a strong type system that ensures type safety and eliminates common programming errors. The type system in Cadence is designed to prevent common errors such as null pointer exceptions and type mismatch errors.
Overall, Cadence is a safe and secure programming language that is designed specifically for smart contracts and DApps on the Flow blockchain. Its resource-oriented programming model and strong type system make it easy to write secure and efficient smart contracts.
Writing Smart Contracts on the Flow Network
To write smart contracts on the Flow network, you will need to have a basic understanding of Cadence. In this section, we will walk you through the steps of writing a simple smart contract on the Flow network.
Creating a New Smart Contract
The first step in writing a smart contract on the Flow network is to create a new smart contract. To do this, you will need to have a development environment set up on your computer. There are several development environments that you can use to write smart contracts on the Flow network, including Visual Studio Code and Atom.
Once you have your development environment set up, you can create a new smart contract file. In Visual Studio Code, you can create a new file by clicking on the “New File” button in the explorer pane. In Atom, you can create a new file by clicking on the “New File” button in the file tree.
In your new smart contract file, you will need to define the contract using the Cadence syntax. The basic syntax for defining a smart contract in Cadence is as follows:
pub contract MyContract {
// Define storage for the contract
var myVariable: Int
// Define a public function
pub fun getMyVariable(): Int {
return myVariable
}
// Define a public function that sets the value of myVariable
pub fun setMyVariable(newValue: Int) {
myVariable = newValue
}
}
In this example, we are defining a new smart contract called “MyContract”. We are defining a storage variable called “myVariable”, which is of type Int. The contract also includes two public functions: “getMyVariable” and “setMyVariable”.
The “getMyVariable” function is a read-only function that returns the current value of “myVariable”. The function does not modify the state of the contract.
The “setMyVariable” function, on the other hand, is a function that modifies the state of the contract by setting the value of “myVariable” to the input parameter “newValue”.
Data Types
Like Solidity, Cadence has a number of data types for variables. Some of the data types include:
Int
- signed integer of arbitrary sizeUInt
- unsigned integer of arbitrary sizeBool
- Boolean valuestrue
andfalse
String
- text string of arbitrary lengthAddress
- a 20-byte Ethereum-style addressCharacter
- a single UTF-8 characterArray
- a list of elements of the same typeDictionary
- a collection of key-value pairs where the keys are of one type and the values are of another type
Flow Playground
Flow Playground is an online code editor that allows you to write, test, and deploy Cadence smart contracts without having to set up a local development environment. It’s a great way to get started with Cadence.
To use the Flow Playground, you’ll need to create an account with Flow. Once you’ve created an account, you can access the Flow Playground and create a new project. In the project, you can create a new file for your Cadence smart contract.
Here’s an example of a simple smart contract in Cadence that stores a value and allows it to be retrieved:
In this smart contract, we declare a public variable called value
to store an integer value. We also have two public functions, setValue
and getValue
, that allow us to set and retrieve the value.
Deploying Cadence Smart Contracts
To deploy a Cadence smart contract, you’ll need to have a Flow account and set up a development environment. You’ll also need to install the Flow CLI, which is a command-line interface tool for interacting with the Flow blockchain.
Once you’ve set up your development environment and installed the Flow CLI, you can deploy your smart contract to the Flow blockchain using the following command:
flow project deploy --network NETWORK_NAME
Writing and Deploying a Smart Contract on Flow
Now that we’ve covered the basics of Cadence and smart contract programming on the Flow blockchain, let’s walk through the process of writing and deploying a simple smart contract on Flow.
For this example, we’ll create a simple contract that allows users to store and retrieve a string message.
First, we’ll create a new project directory and initialize it with npm.
mkdir my-project
cd my-project
npm init -y
Next, we’ll install the Flow CLI and create a new Flow project.
npm install -g @onflow/cli
flow init
This will create a new Flow project with a flow.json
file, which specifies the network configuration and contract paths.
Now, let’s create a new contract file called Message.cdc
in the cadence
directory.
mkdir cadence
touch cadence/Message.cdc
We’ll define a simple contract that stores and retrieves a message.
// cadence/Message.cdc
pub contract Message {
// Define a public field to store the message
pub var message: String
// A public function to set the message
pub fun setMessage(_message: String) {
self.message = _message
}
// A public function to get the message
pub fun getMessage(): String {
return self.message
}
}
This contract defines a public field message
to store the message, and two public functions setMessage
and getMessage
to set and retrieve the message respectively.
Next, we’ll deploy the contract to the Flow emulator for testing. First, we need to start the emulator.
flow emulator start
This will start the emulator at localhost:3569
.
Next, we’ll deploy the contract using the Flow CLI.
flow project deploy --network emulator
This will compile and deploy the contract to the emulator. The CLI will output the contract address, which we’ll use to interact with the contract.
Now, let’s interact with the contract using the Flow CLI.
First, we’ll set a message using the setMessage
function.
flow transactions send ./cadence/transactions/set-message.cdc --signer emulator-account
This will send a transaction to the contract to set the message. The CLI will prompt us to enter the message.
Next, we’ll retrieve the message using the getMessage
function.
flow scripts execute ./cadence/scripts/get-message.cdc --signer emulator-account
This will execute a script to retrieve the message. The CLI will output the message.
Congratulations! You’ve just written and deployed a simple smart contract on the Flow blockchain using Cadence.
Conclusion
In this article, we’ve covered the basics of Cadence and smart contract programming on the Flow blockchain. We’ve discussed the benefits of using Cadence for smart contract programming and explored the key concepts and syntax of the language. We’ve also walked through the process of writing and deploying a simple smart contract on Flow.
As the Flow ecosystem continues to grow, Cadence is becoming an increasingly important tool for developers looking to build decentralized applications on the platform. Whether you’re a seasoned blockchain developer or just getting started with smart contract programming, learning Cadence is a valuable skill to have in your toolkit.
We hope this crash course has provided you with a solid foundation in Cadence and smart contract programming on Flow. Happy coding!
Tags:
Latest Articles
Stay up-to-date with the latest industry trends and insights by reading articles from our technical experts, providing expertise on cutting-edge technologies in the crypto and fintech space.