4 minutes
A Comprehensive Smart Contract Programming Crash Course on the Polkadot Blockchain: A Guide for Software Developers
In this article, we will provide a comprehensive crash course on smart contract programming on the Polkadot blockchain. This guide is geared towards software developers who are familiar with programming languages such as Solidity and JavaScript.
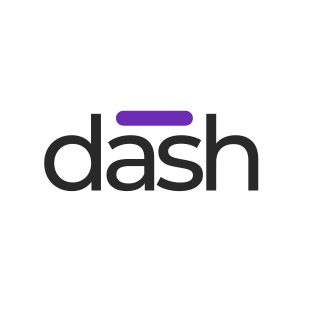
Purple Dash
21/05/2023 5:47 AMTable of Contents
Smart contracts are self-executing programs that run on a blockchain. They allow for the creation of decentralized applications (DApps) that can perform various functions, such as transferring tokens, enforcing rules, and executing complex financial transactions. In this article, we will provide a comprehensive crash course on smart contract programming on the Polkadot blockchain. This guide is geared towards software developers who are familiar with programming languages such as Solidity and JavaScript.
1. Getting Started with Polkadot
Polkadot is a blockchain network that allows for interoperability between different blockchains. It uses a unique architecture called “parachains,” which are independent blockchains that are connected to the Polkadot network. Developers can create their own parachains and customize them to their specific needs.
To get started with Polkadot, you will need to install the Polkadot JS extension on your browser. This extension allows you to interact with the Polkadot network and deploy smart contracts.
2. Smart Contract Languages
Polkadot supports multiple smart contract languages, including Rust, Ink!, and Solidity. Rust is a systems programming language that is known for its performance and security. Ink! is a new smart contract language that is specifically designed for Polkadot. Solidity is a popular smart contract language that is used on the Ethereum blockchain.
In this article, we will focus on Ink!, which is a relatively new language that is gaining popularity among Polkadot developers. Ink! is a Rust-based language that is optimized for building smart contracts on Polkadot.
3. Ink! Smart Contracts
Ink! smart contracts are written in Rust and compiled into WebAssembly (Wasm) bytecode. Ink! provides a set of macros and libraries that make it easy to write smart contracts and interact with the Polkadot network.
To get started with Ink!, you will need to install the Ink! CLI. This CLI provides a set of tools that allow you to create, compile, and deploy Ink! smart contracts.
Let’s take a look at a simple Ink! smart contract that allows users to store and retrieve data on the blockchain.
use ink_lang::contract;
#[contract]
mod storage {
#[ink(storage)]
pub struct Storage {
value: i32,
}
impl Storage {
#[ink(constructor)]
pub fn new(&mut self, init_value: i32) {
self.value = init_value;
}
#[ink(message)]
pub fn get(&self) -> i32 {
self.value
}
#[ink(message)]
pub fn set(&mut self, new_value: i32) {
self.value = new_value;
}
}
}
In this smart contract, we define a Storage struct that contains a single field called “value.” We also define three functions: a constructor function that initializes the value field, a get function that returns the current value, and a set function that allows users to update the value.
To compile and deploy this smart contract, you can use the Ink! CLI:
$ ink new storage
$ ink build
$ ink test
$ ink deploy
4. Interacting with Smart Contracts
Once you have deployed a smart contract on the Polkadot network, you can interact with it using various tools and libraries. The most common way to interact with a smart contract is through a Polkadot JS app that allows you to send transactions and read data from the contract.
Let’s take a look at how we can interact with the Storage smart contract that we defined earlier.
import { ApiPromise, WsProvider } from "@polkadot/api";
import { Keyring } from "@polkadot/keyring";
import { ContractPromise } from "@polkadot/api-contract";
async function main() {
const wsProvider = new WsProvider("ws://localhost:9944");
const api = await ApiPromise.create({ provider: wsProvider });
const keyring = new Keyring({ type: "sr25519" });
const alice = keyring.addFromUri("//Alice");
const contractAddress = "5G1qJrKkMzK5KPVBgKP3ApjUu62qdWUVaGAti1sR8GRqTUoe";
const contract = new ContractPromise(api, abi, contractAddress);
const value = await contract.query.get(alice.address, { value: 0 });
console.log("Current value: ", value.output.toHuman());
await contract.tx.set(42, { value: 1000000000000, gasLimit: -1 }).signAndSend(alice);
const newValue = await contract.query.get(alice.address, { value: 0 });
console.log("New value: ", newValue.output.toHuman());
}
main();
In this example, we use the Polkadot JS API to connect to a local Polkadot node and retrieve the current value of the Storage contract. We then update the value using the set function and sign and send a transaction to the network. Finally, we retrieve the new value of the contract to confirm that the update was successful.
5. Smart Contract Security
Smart contract security is a critical aspect of blockchain development. Smart contracts are immutable, which means that once they are deployed, they cannot be modified or deleted. This makes it essential to ensure that smart contracts are secure and free from vulnerabilities that can be exploited by attackers.
Polkadot provides several tools and best practices to help developers ensure the security of their smart contracts. One of the most important tools is the Rust programming language, which is known for its memory safety and security features. Rust makes it easier to write secure code that is less prone to vulnerabilities such as buffer overflows and memory leaks.
Another important security best practice is to use standard libraries and frameworks that have been thoroughly tested and audited by the community. Polkadot provides a set of built-in libraries and frameworks that are optimized for building secure smart contracts.
Conclusion
In this article, we provided a comprehensive crash course on smart contract programming on the Polkadot blockchain. We covered topics such as the Polkadot architecture, Ink! smart contracts, interacting with smart contracts, and smart contract security. We also provided several code examples to help developers get started with Polkadot smart contract development.
Polkadot is an exciting new blockchain network that provides unique features and capabilities for decentralized application development. With its support for multiple smart contract languages and its focus on security, Polkadot is well-positioned to become a leading platform for smart contract development in the years to come.
Latest Articles
Stay up-to-date with the latest industry trends and insights by reading articles from our technical experts, providing expertise on cutting-edge technologies in the crypto and fintech space.