5 minutes
Exploring the Power of Soul Bound Tokens: A Software Engineer’s Guide to Creating Meaningful NFTs on the Blockchain
In this article, we’ll dive deeper into the technical aspects of soul bound tokens and explore some potential use cases for this exciting new technology.
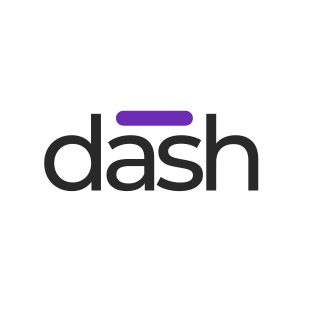
Purple Dash
15/07/2023 5:13 AMTable of Contents
As the world of blockchain technology continues to grow, new and innovative use cases for blockchain are constantly being developed. One of these use cases is the concept of “soul bound tokens,” a unique type of token that is becoming increasingly popular in the blockchain space. As a software engineer, it’s important to understand what soul bound tokens are, how they work, and how they can be implemented in various applications. In this article, we’ll dive deeper into the technical aspects of soul bound tokens and explore some potential use cases for this exciting new technology.
What are soul bound tokens?
Soul bound tokens are a type of non-fungible token (NFT) that are uniquely tied to a specific user or account. Unlike traditional NFTs, which can be bought, sold, and traded freely, soul bound tokens are “soulbound” to their original owner and cannot be transferred to another user or account.
The idea behind soul bound tokens is to create a sense of ownership and exclusivity. By tying a token to a specific user, it creates a special bond between the user and the token. This can be particularly valuable for digital assets that have sentimental or emotional value, such as virtual pets or collectibles.
How do soul bound tokens work?
Soul bound tokens are created using smart contracts on a blockchain platform, such as Ethereum. When a user creates a soul bound token, they must specify the account that the token is soulbound to. This is typically done by including the account address as a parameter in the smart contract.
Once the soul bound token is created, it is “locked” to the specified account. This means that the token cannot be transferred to another account or user, and can only be accessed by the original owner. The smart contract ensures that the token remains soulbound by validating that the user accessing the token is the same user that the token is tied to.
To illustrate how soul bound tokens work in practice, let’s take a look at a simple smart contract implementation. In this example, we’ll create a soul bound token for a virtual pet, where the token is tied to the user’s Ethereum account.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract VirtualPet is ERC721URIStorage, Ownable {
uint256 private _tokenIdCounter;
constructor() ERC721("VirtualPet", "VPET") {}
function mint(address to, string memory tokenURI) public onlyOwner returns (uint256) {
_tokenIdCounter++;
uint256 tokenId = _tokenIdCounter;
_mint(to, tokenId);
_setTokenURI(tokenId, tokenURI);
_lockToken(to, tokenId);
return tokenId;
}
function _lockToken(address account, uint256 tokenId) internal {
require(_isApprovedOrOwner(account, tokenId), "SoulboundToken: caller is not owner nor approved");
_lockToken(tokenId);
}
function _lockToken(uint256 tokenId) internal {
_tokenApprovals[tokenId] = address(this);
}
function unlockToken(uint256 tokenId) public {
require(_isApprovedOrOwner(msg.sender, tokenId), "SoulboundToken: caller is not owner nor approved");
_unlockToken(tokenId);
}
function _unlockToken(uint256 tokenId) internal {
delete _tokenApprovals[tokenId];
}
function isLocked(uint256 tokenId) public view returns (bool) {
return _tokenApprovals[tokenId] == address(this);
}
}
In this smart contract, we’ve created a simple implementation of a soulbound token for a virtual pet. The VirtualPet
contract extends the ERC721URIStorage
contract from the OpenZeppelin library, which provides the standard implementation for NFTs with metadata. We also import the Ownable
contract, which allows us to restrict access to certain functions to the contract owner.
The VirtualPet
contract includes a mint
function, which creates a new soul bound token and locks it to the specified account. When a new token is minted, we increment a _tokenIdCounter
variable, assign the new ID to tokenId
, and then call the _mint
function from the ERC721URIStorage
contract to mint the new token to the specified address. We also set the token's metadata using the _setTokenURI
function.
After the token is minted, we call the _lockToken
function to lock the token to the specified account. This is done by setting the token approval to the contract address, which prevents it from being transferred to another account. The _lockToken
function is called internally, and is only accessible by the contract owner.
To unlock the token, we provide a public unlockToken
function that can be called by the token owner. This function checks that the caller is the token owner or has been approved by the owner, and then calls the _unlockToken
function to unlock the token. The _unlockToken
function simply deletes the token approval, allowing the token to be transferred again.
Finally, we provide a isLocked
function that can be used to check whether a token is currently locked to the contract address.
Use cases for soul bound tokens
Soul bound tokens have a wide range of potential use cases, particularly in applications where digital assets have sentimental or emotional value. Here are a few examples of how soul bound tokens could be used in different contexts:
Gaming
Soul bound tokens could be used in gaming applications to create unique virtual assets that are tied to a player’s account. For example, a game developer could create a soul bound token for a rare in-game item, such as a legendary sword or mount. Once a player acquires the token, it becomes a permanent part of their account and cannot be traded or sold. This creates a sense of ownership and exclusivity for the player, and could be used to incentivize players to participate in certain activities or events within the game.
Collectibles
Soul bound tokens could also be used in collectible applications, such as digital art or trading cards. In this context, the soul bound token represents a unique version of the collectible that is tied to the owner’s account. For example, an artist could create a soul bound token for a limited edition digital art piece, with each token representing a unique version of the artwork. Once a user purchases a token, they become the exclusive owner of that version of the artwork and can display it as part of their collection.
Identity verification
Soul bound tokens could be used as a form of identity verification, particularly in decentralized applications. For example, a user could create a soul bound token that is tied to their real-world identity, such as their passport or driver’s license. Once the token is created, it becomes a permanent part of the user’s account and cannot be transferred or sold. This creates a strong link between the user’s blockchain identity and their real-world identity, which could be useful in a range of applications, such as online voting or financial services.
Conclusion
Soul bound tokens are a unique type of NFT that are becoming increasingly popular in the blockchain space. By tying a token to a specific user or account, soul bound tokens create a sense of ownership and exclusivity that can be valuable in a range of applications. As a software engineer, it’s important to consider the implications of using soul bound tokens in your applications. While they can add an interesting dynamic to your project, they also introduce additional complexity that needs to be managed. Here are a few considerations to keep in mind:
Security
Because soul bound tokens are locked to a specific account, it’s important to ensure that the locking mechanism is secure and cannot be easily circumvented. You should also consider how you will handle situations where a user loses access to their account or has their account compromised.
User experience
Soul bound tokens can be a powerful tool for creating a sense of ownership and exclusivity, but they can also be frustrating for users who want to trade or sell their tokens. You should carefully consider whether a soul bound token is the right choice for your application, and if so, how you can mitigate any negative user experiences.
Scalability
As with any blockchain application, scalability is an important consideration when working with soul bound tokens. Because each token is tied to a specific account, it’s important to ensure that your locking mechanism can handle large numbers of users without impacting performance.
Despite these challenges, soul bound tokens offer an exciting opportunity for software engineers to create unique and compelling applications on the blockchain. By leveraging the power of NFTs and blockchain technology, we can create digital assets that have real emotional and sentimental value for users. As the blockchain space continues to evolve, it will be exciting to see how developers use soul bound tokens to push the boundaries of what’s possible on the blockchain.
Tags:
Latest Articles
Stay up-to-date with the latest industry trends and insights by reading articles from our technical experts, providing expertise on cutting-edge technologies in the crypto and fintech space.