14 minutes
Unlocking the Potential: Advanced Smart Contract Programming with Ink!
Aimed at software developers, we will delve into Ink!’s programming language basics, demonstrate the construction of complex smart contracts, highlight security best practices, and provide insights into the tools and resources available for Ink! smart contract development.
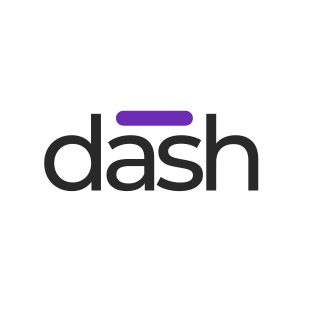
Purple Dash
15/07/2023 4:29 AMTable of Contents
As blockchain technology continues to revolutionize various industries, smart contracts have emerged as a cornerstone of decentralized applications. With their ability to automate and execute agreements without intermediaries, smart contracts offer transparency, efficiency, and trust. In the realm of smart contract programming, Ink! has gained prominence as a language specifically designed for building secure and efficient contracts on the Polkadot network. In this article, we will explore the world of advanced smart contract programming with Ink!. Aimed at software developers, we will delve into Ink!’s programming language basics, demonstrate the construction of complex smart contracts, highlight security best practices, and provide insights into the tools and resources available for Ink! smart contract development. By the end, developers will have a solid foundation to harness the full potential of Ink! and unlock the possibilities of building advanced decentralized applications.
Understanding Ink! Programming Language Basics
In this section, we will dive into the fundamental concepts of the Ink! programming language. We’ll explore the syntax, data types, control flow, event handling, error management, and testing in Ink! through code examples and detailed explanations.
A. Syntax and Structure of Ink! Smart Contracts
Ink! follows a syntax that is similar to other popular programming languages, making it relatively easy for developers to get started. Let’s take a look at a simple Ink! smart contract:
contract MyContract {
// State variable
storage value: i32;
// Constructor
constructor() {
value = 0;
}
// Public function to set the value
pub(external) fn set_value(&mut self, new_value: i32) {
value = new_value;
}
// Public view function to get the value
pub(external) fn get_value(&self) -> i32 {
value
}
}
The contract
keyword is used to define a smart contract called MyContract
.
- The
storage
keyword indicates a state variable,value
, which will persist between function calls. - The
constructor
is a special function that initializes the contract's state when it is deployed. - The
pub(external)
modifier indicates that the following functions can be called from outside the contract. - The
set_value
function modifies the value ofvalue
based on the providednew_value
. - The
get_value
function retrieves the current value ofvalue
and returns it.
B. Variables, Data Types, and Control Flow in Ink!
Ink! supports various data types, including integers, booleans, strings, arrays, and more. Let’s see some examples:
// Integer variable
let my_number: i32 = 42;
// Boolean variable
let is_valid: bool = true;
// String variable
let my_string: String = String::from("Hello, world!");
// Array
let numbers: [i32; 3] = [1, 2, 3];
// Control flow - if-else statement
if my_number > 50 {
ink_env::println("Number is greater than 50");
} else {
ink_env::println("Number is less than or equal to 50");
}
We declare an integer variable my_number
and assign it the value 42
.
- The boolean variable
is_valid
is assigned the valuetrue
. - A string variable
my_string
is created using theString::from
function. - We define an array
numbers
with three elements: 1, 2, and 3. - The if-else statement checks if
my_number
is greater than 50 and prints the corresponding message.
C. Handling Events and Messages in Ink!
Events play a vital role in smart contract development to communicate significant occurrences. Let’s see how we can define events and handle them in Ink!:
event ValueSet(i32);
contract MyContract {
// ...
pub(external) fn set_value(&mut self, new_value: i32) {
value = new_value;
self.env().emit_event(ValueSet(new_value));
}
}
We define an event ValueSet
that takes an i32
parameter.
- Inside the
set_value
function, after updating thevalue
, we emit theValueSet
event with the new value as an argument.
D. Error Handling and Exception Management in Ink!
Ink! provides mechanisms for handling errors and exceptions. Let’s explore the usage of try-catch blocks:
pub(external) fn divide_numbers(&self, a: i32, b: i32) -> Result<i32, String> {
if b == 0 {
Err(String::from("Division by zero"))
} else {
Ok(a / b)
}
}
pub(external) fn perform_division(&self) {
let result = match self.divide_numbers(10, 0) {
Ok(value) => value,
Err(err) => {
ink_env::println(&err);
return;
}
};
ink_env::println("Result: ", result);
}
The divide_numbers
function takes two integers as input and returns a Result
type, where Ok
represents a successful result and Err
represents an error.
- Inside the
perform_division
function, we use a match statement to handle the result of thedivide_numbers
function. If division by zero occurs, an error message is printed, and the function returns.
E. Testing and Debugging Ink! Smart Contracts
Testing and debugging are crucial aspects of smart contract development. Ink! provides tools and frameworks for testing and debugging smart contracts, ensuring their correctness and robustness. Writing comprehensive unit tests is important to validate the contract’s functionality and catch potential bugs or vulnerabilities.
To facilitate testing, Ink! provides testing frameworks such as ink_lang_test and ink_env::test. Debugging techniques, such as printing debug statements using ink_env::println
, can help identify and resolve issues effectively during the development process.
By understanding the syntax, data types, control flow, event handling, error management, and testing capabilities of Ink!, developers can effectively build secure and efficient smart contracts on the Polkadot network.
Advanced Concepts in Smart Contract Programming
A. Contract Lifecycle and Instantiation in Ink!
Ink! provides a built-in contract lifecycle management system that allows developers to control the creation and initialization of contracts. Let’s explore the contract lifecycle and instantiation process using code examples:
contract MyContract {
// Constructor
#[ink(constructor)]
fn new() -> Self {
Self {}
}
// Initialization function
#[ink(message)]
fn initialize(&mut self, param1: u32, param2: String) {
// Initialization logic here
}
}
- The
new
function is annotated with#[ink(constructor)]
, indicating that it serves as the constructor for the contract. It is called when a new instance of the contract is created. - The
initialize
function is annotated with#[ink(message)]
, allowing it to be called after the contract is instantiated. It is used to initialize the contract's state with the provided parameters.
B. Event Handling and Logging in Smart Contracts
Event handling is a crucial aspect of smart contract development as it allows contracts to emit and capture important occurrences. In Ink!, events are defined using structures and can be emitted within contract functions. Let’s see an example:
event ValueSet(u32);
contract MyContract {
// ...
#[ink(message)]
fn set_value(&mut self, new_value: u32) {
// Set the value
self.value = new_value;
// Emit the ValueSet event
self.env().emit_event(ValueSet(new_value));
}
}
- We define an event called
ValueSet
that takes au32
parameter. - Inside the
set_value
function, after updating thevalue
, we emit theValueSet
event with the new value as an argument usingself.env().emit_event
.
C. Error Handling and Exception Management in Ink!
Ink! provides mechanisms for handling errors and exceptions, ensuring the reliability and security of smart contracts. Let’s explore the usage of error handling and exception management in Ink!:
#[ink(message)]
fn divide_numbers(&self, a: u32, b: u32) -> Result<u32, String> {
if b == 0 {
return Err(String::from("Division by zero"));
}
Ok(a / b)
}
#[ink(message)]
fn perform_division(&self) {
match self.divide_numbers(10, 0) {
Ok(value) => {
// Handle successful division
}
Err(err) => {
// Handle error
ink_env::println(&err);
}
}
}
- The
divide_numbers
function takes twou32
values as input and returns aResult
type, whereOk
represents a successful result, andErr
represents an error. - Inside the
perform_division
function, we use a match statement to handle the result of thedivide_numbers
function. If division by zero occurs, an error message is printed.
By understanding and utilizing the advanced concepts in smart contract programming, such as contract lifecycle and instantiation, event handling and logging, and error handling and exception management, developers can build more sophisticated and robust Ink! smart contracts that meet specific requirements and ensure reliable execution.
Building Complex Smart Contracts with Ink!
A. Writing Reusable and Modular Code with Ink!
Ink! empowers developers to write reusable and modular code, allowing for better code organization and easier maintenance. Let’s explore how to achieve code modularity in Ink! with an example:
contract MathOperations {
#[ink(message)]
pub fn add(&self, a: u32, b: u32) -> u32 {
a + b
}
#[ink(message)]
pub fn subtract(&self, a: u32, b: u32) -> u32 {
a - b
}
}
contract MyContract {
math: MathOperations,
#[ink(constructor)]
pub fn new() -> Self {
Self {
math: MathOperations::new(),
}
}
#[ink(message)]
pub fn perform_operations(&self, a: u32, b: u32) -> u32 {
let sum = self.math.add(a, b);
let difference = self.math.subtract(a, b);
sum * difference
}
}
- We define a separate contract called
MathOperations
to encapsulate mathematical operations. - The
MathOperations
contract contains two public functions,add
andsubtract
, for performing addition and subtraction operations, respectively. - In the
MyContract
contract, we create an instance ofMathOperations
namedmath
to access its functions. - The
perform_operations
function demonstrates how the functions fromMathOperations
can be utilized withinMyContract
.
B. Implementing Access Control and Permission Management
Access control and permission management are crucial aspects of smart contract development. Ink! provides flexibility in implementing access control mechanisms. Let’s explore an example of implementing access control using modifier-like functions:
contract MyContract {
owner: AccountId,
#[ink(constructor)]
pub fn new(owner: AccountId) -> Self {
Self { owner }
}
#[ink(message)]
pub fn perform_action(&self) {
self.check_owner();
// Perform action only allowed for the contract owner
}
#[ink(message)]
pub fn transfer_ownership(&mut self, new_owner: AccountId) {
self.check_owner();
self.owner = new_owner;
}
#[ink(message)]
pub fn check_owner(&self) {
assert_eq!(self.env().caller(), self.owner, "Unauthorized access");
}
}
- The
MyContract
contract has anowner
variable to store the address of the contract owner. - The
new
constructor takes anowner
parameter and initializes theowner
variable. - The
perform_action
function andtransfer_ownership
function both call thecheck_owner
function to ensure that only the contract owner can perform certain actions or transfer ownership. - The
check_owner
function compares the caller's address with the contract owner's address, and if they don't match, an assertion error is thrown.
C. Interacting with External Contracts and Oracles
Ink! enables seamless interaction with external contracts and oracles, expanding the capabilities of smart contracts. Let’s explore an example of interacting with an external contract:
#[ink::contract]
mod my_contract {
#[ink(storage)]
pub struct MyContract {
external_contract: ExternalContract,
}
#[ink(constructor)]
pub fn new(external_contract: AccountId) -> Self {
let external_contract = ExternalContract::new(external_contract);
Self {
external_contract,
}
}
#[ink(message)]
pub fn call_external_contract(&self, value: u32) {
self.external_contract.some_function(value);
}
}
#[ink::contract]
mod external_contract {
#[ink(storage)]
pub struct ExternalContract {
owner: AccountId,
}
#[ink(constructor)]
pub fn new(owner: AccountId) -> Self {
Self { owner }
}
#[ink(message)]
pub fn some_function(&self, value: u32) {
// Function logic
}
}
- We define two separate contracts,
MyContract
andExternalContract
. - The
MyContract
contract has a storage variable,external_contract
, which is an instance of theExternalContract
. - The
new
constructor ofMyContract
takes anexternal_contract
parameter and initializes theexternal_contract
variable. - The
call_external_contract
function inMyContract
can call thesome_function
function inExternalContract
using theexternal_contract
instance.
By utilizing code modularity, implementing access control, and interacting with external contracts and oracles, developers can build more complex and versatile smart contracts with Ink! that integrate with other contracts and external data sources, expanding the possibilities of decentralized applications.
Security Best Practices in Ink! Smart Contract Programming
A. Common Vulnerabilities and Pitfalls in Smart Contract Programming
Smart contract programming requires careful attention to security to protect against potential vulnerabilities. Let’s explore some common vulnerabilities and pitfalls and how to address them:
- Reentrancy Attacks: Reentrancy attacks occur when a contract’s function can be maliciously called again before the previous execution completes. To prevent this, use the “Checks-Effects-Interactions” pattern, where critical state changes are performed before interacting with other contracts.
- Integer Overflow and Underflow: Integer overflow and underflow can lead to unexpected behavior and exploitation. Ensure proper range checks and use safe math libraries or built-in language features to prevent these vulnerabilities.
- Unchecked External Calls: External calls to untrusted contracts can introduce risks. Validate inputs, use checks for contract validity, and employ defensive programming techniques when interacting with external contracts.
- Access Control Issues: Incorrect access control mechanisms can lead to unauthorized actions. Implement proper access control logic, restrict sensitive functions, and avoid relying solely on the
caller
provided by the environment.
B. Importance of Code Review and Auditing
Thorough code review and auditing are critical steps in ensuring the security and reliability of smart contracts. Engaging independent auditors or conducting self-audits can help identify vulnerabilities. Consider the following practices:
- Peer Code Review: Involve multiple developers to review and provide feedback on the codebase. This can help identify logical errors, vulnerabilities, and potential improvements.
- Formal Verification: Employ formal verification tools to mathematically prove the correctness of the contract’s behavior against specified properties.
- Independent Auditing: Seek external experts to conduct comprehensive security audits. They can assess the contract’s codebase, logic, and design to identify potential vulnerabilities.
C. Secure Coding Practices and Defensive Programming in Ink!
Adopting secure coding practices and employing defensive programming techniques can significantly enhance the security of Ink! smart contracts. Consider the following recommendations:
- Input Validation and Sanitization: Validate and sanitize all inputs to prevent unexpected behavior or malicious exploitation. Check input ranges, type constraints, and perform thorough validation checks.
- Proper Error Handling: Implement comprehensive error handling and exception management mechanisms. Gracefully handle errors and provide informative error messages to users to aid in debugging and prevent unintended consequences.
- Minimize External Dependencies: Reduce reliance on external contracts, libraries, or oracles to minimize potential attack vectors. Thoroughly assess and review any third-party code used in the contract.
- Immutable Contracts: Once deployed, smart contracts on the blockchain are immutable. Therefore, it is crucial to carefully design, test, and review contracts before deployment to avoid irreversible mistakes or vulnerabilities.
- Regular Updates and Patching: Monitor security updates and vulnerabilities in the Ink! ecosystem. Promptly update contracts when necessary and apply patches or fixes to mitigate potential risks.
By following these security best practices, conducting thorough code reviews, and practicing defensive programming techniques, developers can enhance the security and reliability of their Ink! smart contracts, reducing the risk of vulnerabilities and ensuring the trustworthiness of decentralized applications.
Advanced Ink! Smart Contract Examples
Example 1: Creating a Decentralized Marketplace using Ink!
Contract Design and Architecture:
contract Marketplace {
struct Product {
uint256 id;
address seller;
string name;
uint256 price;
}
Product[] public products;
function createProduct(string memory _name, uint256 _price) public {
uint256 id = products.length + 1;
products.push(Product(id, msg.sender, _name, _price));
}
function getProductsCount() public view returns (uint256) {
return products.length;
}
}
- The
Marketplace
contract defines aProduct
struct to represent the details of a product. - The
products
array holds all the products listed on the marketplace. - The
createProduct
function allows sellers to create a new product by specifying its name and price. - The
getProductsCount
function retrieves the total count of products listed on the marketplace.
Implementing Listing, Buying, and Dispute Resolution Functionality:
contract Marketplace {
// ...
mapping(uint256 => address) public productToBuyer;
function buyProduct(uint256 _productId) public payable {
require(_productId > 0 && _productId <= products.length, "Invalid product ID");
Product storage product = products[_productId - 1];
require(msg.value >= product.price, "Insufficient funds");
productToBuyer[_productId] = msg.sender;
product.seller.transfer(msg.value);
}
function resolveDispute(uint256 _productId, bool _refund) public {
require(_productId > 0 && _productId <= products.length, "Invalid product ID");
require(productToBuyer[_productId] == msg.sender, "Unauthorized");
Product storage product = products[_productId - 1];
if (_refund) {
payable(product.seller).transfer(product.price);
}
}
}
- The
buyProduct
function allows a buyer to purchase a product by providing the product ID and the required payment. - The function verifies that the product ID is valid and the payment amount is sufficient. It transfers the payment to the seller and records the buyer’s address for the product.
- The
resolveDispute
function enables buyers to resolve disputes by requesting a refund from the seller. Only the buyer of a specific product can initiate a dispute resolution.
Example 2: Building a Decentralized Voting System with Ink!
Designing the Voting Contract:
contract Voting {
struct Candidate {
uint256 id;
string name;
uint256 voteCount;
}
mapping(uint256 => Candidate) public candidates;
mapping(address => bool) public voters;
function addCandidate(uint256 _candidateId, string memory _name) public {
require(!isCandidateExists(_candidateId), "Candidate already exists");
candidates[_candidateId] = Candidate(_candidateId, _name, 0);
}
function vote(uint256 _candidateId) public {
require(isCandidateExists(_candidateId), "Candidate does not exist");
require(!voters[msg.sender], "Already voted");
candidates[_candidateId].voteCount++;
voters[msg.sender] = true;
}
function getCandidateVoteCount(uint256 _candidateId) public view returns (uint256) {
require(isCandidateExists(_candidateId), "Candidate does not exist");
return candidates[_candidateId].voteCount;
}
function isCandidateExists(uint256 _candidateId) internal view returns (bool) {
return candidates[_candidateId].id != 0;
}
}
- The
Voting
contract manages a list of candidates and tracks the vote count for each candidate. - The
Candidate
struct represents a candidate with an ID, name, and vote count. - The
addCandidate
function allows the contract owner to add candidates by providing their ID and name. - The
vote
function enables eligible voters to cast their votes for a specific candidate. - The
getCandidateVoteCount
function retrieves the vote count for a given candidate. - The
isCandidateExists
internal function checks if a candidate exists based on their ID.
Ensuring Transparency and Integrity of the Voting Process:
contract Voting {
// ...
event VoteCasted(address indexed voter, uint256 indexed candidateId);
modifier onlyValidCandidate(uint256 _candidateId) {
require(isCandidateExists(_candidateId), "Invalid candidate");
_;
}
function vote(uint256 _candidateId) public onlyValidCandidate(_candidateId) {
require(!voters[msg.sender], "Already voted");
candidates[_candidateId].voteCount++;
voters[msg.sender] = true;
emit VoteCasted(msg.sender, _candidateId);
}
}
- The
VoteCasted
event is emitted whenever a vote is successfully casted, providing transparency to the voting process. - The
onlyValidCandidate
modifier is used to validate that the provided candidate ID is valid before executing the function. - By emitting the
VoteCasted
event, the contract captures and logs the voter's address and the candidate ID, ensuring the integrity of the voting process.
These advanced examples demonstrate the implementation of a decentralized marketplace and a decentralized voting system using Ink!. The examples highlight the architecture, functionality, and best practices associated with building complex smart contracts on the Polkadot network.
Tools and Resources for Advanced Smart Contract Development with Ink!
A. Development Environments and IDEs for Ink!
When working with Ink! smart contracts, developers can leverage various development environments and integrated development environments (IDEs) to enhance their productivity and code quality. Some popular tools and environments for Ink! development include:
- Polkadot{.js} Apps: Polkadot{.js} Apps is a comprehensive development environment that provides a user-friendly interface for deploying, testing, and interacting with Ink! smart contracts. It offers a powerful set of tools and features, including a built-in Ink! smart contract editor, contract deployment manager, and contract interaction interface.
- Sublime Text with Sublime Ink!: Sublime Ink! is a plugin for the Sublime Text editor that offers syntax highlighting, autocompletion, and code snippets for Ink! smart contract development. It enhances the development experience by providing a more tailored environment for working with Ink! code.
B. Testing Frameworks and Tools for Ink!
Smart Contracts Testing is a critical aspect of smart contract development to ensure correctness and identify vulnerabilities. Ink! provides testing frameworks and tools that aid developers in writing comprehensive tests for their smart contracts. Some notable testing frameworks and tools for Ink! smart contracts include:
- ink_lang_test: ink_lang_test is an official testing framework for Ink! smart contracts. It provides utilities for writing unit tests that cover various scenarios and use cases. Developers can write test cases to verify the behavior and functionality of their contracts, ensuring they work as intended.
- ink_env::test: ink_env::test is a module provided by Ink! that facilitates the simulation of contract execution within a test environment. It allows developers to simulate different contract interactions, test edge cases, and verify contract behavior without deploying to the live network.
C. Ink! Community and Online Resources for Learning and Collaboration
Joining the Ink! community and exploring online resources can greatly aid developers in learning, collaborating, and staying up to date with the latest advancements in Ink! smart contract development. Some valuable resources and communities for Ink! developers include:
- Ink! GitHub Repository: The official Ink! GitHub repository serves as a central hub for Ink! development. It contains documentation, examples, and the source code for the Ink! programming language and related tools. Developers can explore the repository to gain insights, access code samples, and contribute to the project.
- Ink! Subreddit and Forum: The Ink! subreddit and forum provide platforms for developers to engage in discussions, ask questions, share knowledge, and seek guidance from the community. It is an excellent place to connect with fellow developers, learn from their experiences, and collaborate on Ink! projects.
- Ink! Documentation: The official documentation for Ink! offers comprehensive guidance and references for smart contract development using Ink!. It covers topics ranging from language syntax and features to contract deployment and interaction. Developers can refer to the documentation for in-depth explanations, code examples, and best practices.
- Ink! Community Events: Participating in community events, such as hackathons, workshops, and webinars, can provide valuable opportunities to network with other developers, learn from experts, and showcase innovative projects. Stay informed about upcoming Ink! events through community channels and newsletters.
By utilizing the available development environments, testing frameworks, and community resources, developers can enhance their productivity, ensure code quality, and engage in a vibrant community of Ink! smart contract developers. These tools and resources are invaluable in advancing one’s skills and staying at the forefront of Ink! development.
Conclusion
Ink! has emerged as a powerful programming language for advanced smart contract development on the Polkadot network. With its user-friendly syntax, extensive feature set, and robust ecosystem, Ink! enables developers to build secure, scalable, and interoperable smart contracts.
In this article, we explored the world of advanced smart contract programming with Ink!. We delved into understanding Ink! programming language basics, covering topics such as syntax, data types, control flow, event handling, error management, and testing. We then moved on to building complex smart contracts using Ink!, showcasing examples like a decentralized marketplace and a decentralized voting system. These examples highlighted the design principles, implementation strategies, and best practices for creating sophisticated Ink! smart contracts.
Furthermore, we emphasized the significance of security best practices in Ink! smart contract programming. By understanding common vulnerabilities, conducting code reviews, and implementing secure coding practices, developers can mitigate risks and protect against potential exploits.
To aid developers in their Ink! smart contract journey, we discussed tools and resources available. Development environments and IDEs like Polkadot{.js} Apps and Sublime Ink! streamline the development process, while testing frameworks like ink_lang_test and ink_env::test ensure the correctness and reliability of smart contracts. Engaging with the Ink! community and exploring online resources, such as the official Ink! GitHub repository, documentation, and forums, provide avenues for learning, collaboration, and staying updated with the latest advancements in Ink!.
Ink! presents a world of possibilities for building decentralized applications on the Polkadot network. As the Ink! ecosystem continues to evolve, developers have the opportunity to contribute, innovate, and shape the future of smart contract development.
So, whether you’re a seasoned developer or just starting your journey, embracing advanced smart contract programming with Ink! opens doors to a realm of decentralized possibilities, where secure, scalable, and interoperable applications can thrive.
Tags:
Latest Articles
Stay up-to-date with the latest industry trends and insights by reading articles from our technical experts, providing expertise on cutting-edge technologies in the crypto and fintech space.